Implementing AI-Driven Conversational Surveys with OpenAI
This article explores implementing AI-driven conversational surveys using OpenAI's GPT-3. It highlights the benefits, prerequisites, and steps involved, along with code snippets for implementation. Discover how businesses can revolutionize feedback collection and enhance user engagement.
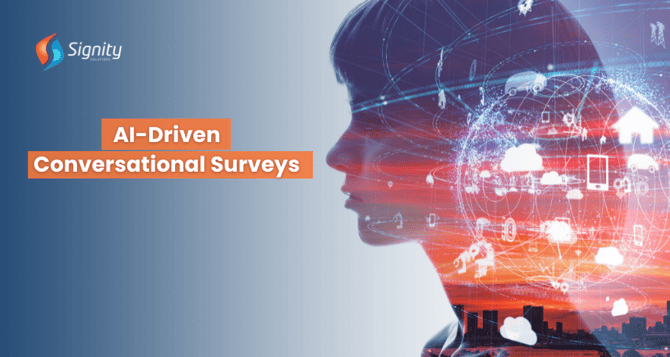
Conversational surveys have gained traction as an effective means of gathering user feedback and data. Unlike traditional surveys that often suffer from low engagement and accuracy, AI-driven conversational surveys offer a more interactive and user-friendly experience. In this article, we'll delve into the process of implementing such surveys using OpenAI's GPT-3 and provide code snippets to help you kickstart your project.
Prerequisites
Before delving into implementation, ensure you have the following prerequisites:
1. OpenAI API Key:
Obtain an API key from OpenAI to access the GPT-3 model. Sign up for access on the OpenAI website.
2. Python Environment:
Python 3 should be installed on your system.
3. OpenAI Python SDK:
Install the OpenAI Python SDK using pip:
pip install openai |
Setting Up the Survey
To create an AI-driven conversational survey, define the questions and prompts you want to ask users. Here's a simple example setup:
survey = [ "Welcome to the survey! We'd like to know more about your preferences.", "What is your favorite color?", "Do you prefer coffee or tea?", "Could you tell us your age?", "Any additional comments or feedback?", ] |
Using the OpenAI GPT-3 Model
Now, let's utilize the OpenAI GPT-3 model to generate responses to these survey prompts. Here's a function to interact with the model:
import openai # Replace 'your-api-key' with your actual API key api_key = 'your-api-key' openai.api_key = api_key def generate_responses(prompts, max_tokens=50): conversation = [] for prompt in prompts: conversation.append({"role": "system", "content": prompt}) response = openai.Completion.create( engine="text-davinci-002", messages=conversation, max_tokens=max_tokens ) return response.choices[0].message["content"] |
Running the Survey
Let's run the survey and collect user responses:
user_responses = [] for i in range(1, len(survey)): user_response = input(generate_responses(survey[i-1:i+1]) + "\nYour response: ") user_responses.append(user_response) print("Survey complete! Here are the user responses:") for i, response in enumerate(user_responses): print(f"Question {i+1}: {response}") |
Customization and Enhancement
Customize the survey by adding more prompts, modifying the conversation flow, or adjusting the GPT-3 parameters. Experiment with different structures to enhance engagement and naturalness. In a production environment, handle errors gracefully, incorporate data validation, and gather user feedback to refine the survey experience.
Transform Ideas into Reality with Our AI Solutions!
From concept to deployment, we bring your vision to life through advanced AI development. Reach out to our experts to discuss your project!
Implementing AI-driven conversational surveys with OpenAI's GPT-3 can revolutionize feedback collection, making it more interactive and engaging. With the provided code snippets, you're well-equipped to create your own AI-powered surveys and gather valuable insights from users.